S3D.grouping
This library provides methods to retrieve particular model elements based on certain criteria.
All grouping code snippets within use the model pictured below.
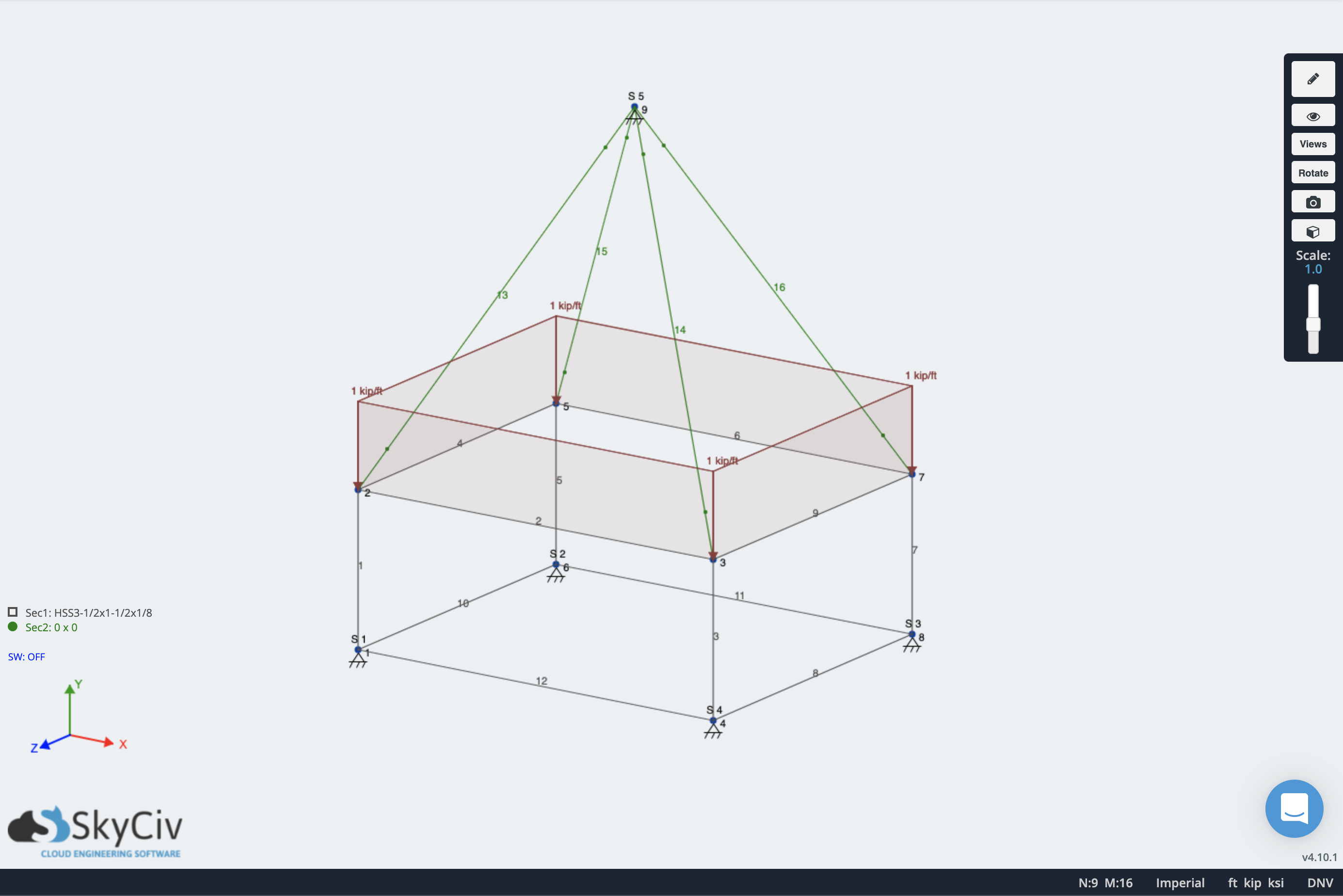
#
NodesbyExtremes
#
Description: Get all nodes at the specified extreme of the model.
Operation Type: Synchronous
Params: S3D.grouping.nodes.byExtremes(Object)
Object properties:
Key | Type | Accepts | Description |
---|---|---|---|
structure | Object | The structure object. | This can be obtained via S3D.structure.get() . |
direction | string | +x , -x , +y , -y , +z , -z . | Direction of the extreme to get. |
unfiltered_ids | [integer] | An array of Node IDs. | If omitted, all nodes in the structure will be checked. |
tol | float | Any normal number. | If the tolerance is 1, and the model length units are in ft, then any nodes within 1ft of the extreme are returned. Defaults to 1e-6 . |
Sample:
byMemberProp
#
Description: Get all nodes that are attached to members that have the specified attribute.
Operation Type: Synchronous
Params: S3D.grouping.nodes.byMemberProp(Object)
Object properties:
Key | Type | Accepts | Description |
---|---|---|---|
structure | Object | The structure object. | This can be obtained via S3D.structure.get() . |
prop | string | A member property. | The member property to check. |
value | Any | Any value. | The value that the member property should be. |
unfiltered_ids | [integer] | An array of member IDs. | Only return members if their ID is included in this array. |
Sample:
byMember
#
Description: Get all nodes that are attached to specified members.
Operation Type: Synchronous
Params: S3D.grouping.nodes.byMember(Object)
Object properties:
Key | Type | Accepts | Description |
---|---|---|---|
structure | Object | The structure object. | This can be obtained via S3D.structure.get() . |
member_ids | [integer] | An array of member IDs. | Get the node IDs of these members. |
unfiltered_ids | [integer] | An array of node IDs. | Only return node IDs if their ID is included in this array. |
Sample:
#
MembersbyNodes
#
Description: Get all members that are attached to any of the given nodes.
Operation Type: Synchronous
Params: S3D.grouping.members.byNodes(Object)
Object properties:
Key | Type | Accepts | Description |
---|---|---|---|
structure | Object | The structure object. | This can be obtained via S3D.structure.get() . |
node_ids | [integer] | An array of node IDs. | If the member uses one of these nodes, it will be included. |
unfiltered_ids | [integer] | An array of member IDs. | Only return members if their ID is included in this array. |
Sample:
bySecName
#
Description: Get all members that have the given section name.
Operation Type: Synchronous
Params: S3D.grouping.members.bySecName(Object)
Object properties:
Key | Type | Accepts | Description |
---|---|---|---|
structure | Object | The structure object. | This can be obtained via S3D.structure.get() . |
section | string | The section name. | All members using this section name will be returned. |
unfiltered_ids | [integer] | An array of member IDs. | Only return members if their ID is included in this array. |
Sample:
byVector
#
Description: Get all members that conform to a specified vector.
Operation Type: Synchronous
Params: S3D.grouping.members.byVector(Object)
Object properties:
Key | Type | Accepts | Description |
---|---|---|---|
structure | Object | The structure object. | This can be obtained via S3D.structure.get() . |
vector | [float] | An X,Y and Z value to define a vector. | All members using this section name will be returned. |
unfiltered_ids | [integer] | Only return members if their ID is included in this array. | |
tol | float | A float representing degrees. | If the angle between the vector and any member is below or equal to this value, it will be returned. Defaults to 1e-6 |
Sample:
byExtremes
#
Description: Get all members at the specified extreme of the model.
Operation Type: Synchronous
Params: S3D.grouping.members.byExtremes(Object)
Key | Type | Accepts | Description |
---|---|---|---|
structure | Object | The structure object. | This can be obtained via S3D.structure.get() . |
direction | string | +x , -x , +y , -y , +z , -z . | Direction of the extreme to get. |
unfiltered_ids | [integer] | An array of Node IDs. | Only return members in this array. If omitted, all members in the structure will be checked. |
both_nodes_on_extreme | boolean | true , false | If both of the member's nodes should be on the extreme. Defaults to false. |
tol | float | Any normal number. | If the tolerance is 1, and the model length units are in ft, then any members within 1ft of the extreme are returned. Defaults to 1e-6 . |
Sample:
#
GeneralbyAttr
#
Description: Get all elements that have the specified attribute.
Operation Type: Synchronous
Params: S3D.grouping.general.byAttr(Object)
Object properties:
Key | Type | Accepts | Description |
---|---|---|---|
structure | Object | The structure object. | This can be obtained via S3D.structure.get() . |
unfiltered_ids | [integer] | An array of element IDs. | Only return elements if their ID is included in this array. |
type | string | nodes , members , materials , supports , etc. | The child array of the structure object to search. |
key | string | Any key inside the iterable objects. | The key to check of each object in the type array. |
value | Any | Any value. | The value to match to the key defined above. |
Sample:
byBounds
#
Description: Get all elements inside a 2D plane. Polygon can be convex or concave. By default it is assumed convex unless specified otherwise. If convex then extra processing is required to triangulate the polygon.
Operation Type: Synchronous
Params: S3D.grouping.general.byBounds(Object)
Object properties:
Key | Type | Accepts | Description |
---|---|---|---|
structure | Object | The structure object. | This can be obtained via S3D.structure.get() . |
unfiltered_ids | [integer] | An array of element IDs. | Only return elements if their ID is included in this array. |
type | string | nodes , members , materials , supports , etc. | The child array of the structure object to search. |
node_ids | [integer] | Nodes IDs to define a 2D polygon. | The key to check of each object in the type array. |
tol | float | Any normal number. | The tolerance to include members outside the polygon. |
concave | boolean | true or false . | Whether the polygon is concave or convex. Defaults true . |
Sample:
byCoord
#
Description: Get all elements that use a node that has a location that meets the specified criteria.
Operation Type: Synchronous
Params: S3D.grouping.general.byCoord(Object)
Object properties:
Key | Type | Accepts | Description |
---|---|---|---|
structure | Object | The structure object. | This can be obtained via S3D.structure.get() . |
unfiltered_ids | [integer] | An array of element IDs. | Only return elements if their ID is included in this array. |
type | string | nodes , members , materials , supports , etc. | The child array of the structure object to search. |
comparison | string | less than , equals to , greater than . | The type of comparison to use when checking a node. |
plane | string | x , y or z . | The plane which to compare in. |
value | float | Any float. | The coordinate to use in the comparison. |
Sample:
byMaterial
#
Description: Get all members that have the given material name.
Operation Type: Synchronous
Params: S3D.grouping.general.byMaterial(Object)
Object properties:
Key | Type | Accepts | Description |
---|---|---|---|
structure | Object | The structure object. | This can be obtained via S3D.structure.get() . |
type | string | nodes , members , materials , supports , etc. | The child array of the structure object to search. |
unfiltered_ids | [integer] | An array of element IDs. | Only return elements if their ID is included in this array. |
material_name | string | The material name. | All elements using this material will be returned. |
Sample:
byPlane
#
Description: Get all elements that are in the specified plane.
Operation Type: Synchronous
Params: S3D.grouping.general.byPlane(Object)
Object properties:
Key | Type | Accepts | Description |
---|---|---|---|
structure | Object | The structure object. | This can be obtained via S3D.structure.get() . |
unfiltered_ids | [integer] | An array of element IDs. | Only return elements if their ID is included in this array. |
type | string | nodes , members , materials , supports , etc. | The child array of the structure object to search. |
node_ids | [integer] | Three node IDs that define a plane. | If an element lies in this plane it will be returned. |
Sample:
byPlaneDetailed
#
Description: An extension of the byPlane
method which returns the element IDs and the perpendicular vector.
Operation Type: Synchronous
Params: S3D.grouping.general.byPlaneDetailed(Object)
Object properties:
Key | Type | Accepts | Description |
---|---|---|---|
structure | Object | The structure object. | This can be obtained via S3D.structure.get() . |
unfiltered_ids | [integer] | An array of element IDs. | Only return elements if their ID is included in this array. |
type | string | nodes , members , materials , supports , etc. | The child array of the structure object to search. |
node_ids | [integer] | Three node IDs that define a plane. | If an element lies in this plane it will be returned. |
Sample:
#
Auto Groupingmembers
#
Description: Members can be automatically grouped by similar attributes. The method takes two parameters - the first parameter is the structure
object and the second is a settings object.
Operation Type: Synchronous
Params: S3D.grouping.autoGrouping.members(Object)
Parameters | Type | Accepts | Description |
---|---|---|---|
structure | Object | The structure object. | This can be obtained via S3D.structure.get() . |
settings | Object | An object of key value pairs. See example below. | Attributes to group by. |
Sample input:
Sample output: