S3D.SB
The S3D.SB
namespace exposes the functionality offered on SkyCiv's Section Builder platform. This includes access to the section database, creating custom sections, analyzing sections and more.
S3D.SB.getLibraryTree
#
Get the list of sections from a specific library.
Key | Type | Accepts | Description |
---|---|---|---|
section_map | array | An array of up to 3 strings | An array defining the keys of the map you wish to return. Use the Section Builder UI for reference. Some examples: ["American"] - will return all American sections ["American", "AISC"] - will return all sections from AISC library["American", "AISC", "W shapes"] - will return all W-Shapes ["Australian", "Cold Formed Steel (Lysaght)", "Angles"] ["Canadian", "CISC", "M Shapes"] - will return all M-Shapes from CISC catalog |
S3D.SB.getLibraryTree
function#
Sample input for the S3D.SB.getLibraryTree
S3D.SB.getLibraryTree
function#
Sample response for the S3D.SB.buildCustomShape
#
SkyCiv Section Builder uses a powerful FEM solver to calculate section properties such as area, moment of inertia, centroids, section moduli, shear properties and torsion properties. Users can use the API to calculate these properties via custom points and line data using S3D.SB.buildCustomShape
.
Key | Type | Accepts | Description |
---|---|---|---|
type | string | points , line | The type of custom shape being built, either: • points - a shape defined by 2 points and the radius;• line - 2 points and the line thickness. |
units | string | mm or metric , in or imperial | To select units system used in Section Builder: • metric - (mm)-distance, (kN)-force, (kN-m)-moment ;• imperial - (in)-distance, (kip)-force, (kip-ft)-moment; Metric system used if the field is undefined. |
points | [[number]] | An array of number arrays | Array of points. For "type": "points" , this is in format [x, y, radius]For "type": "line" , this is in the format [x, y] |
fillet | number | a positive number | The fillet radius in mm |
thickness | number | a positive number | The section thickness in mm |
run_solve | boolean | true , false | If solve should be executed following the build. |
material_id | integer | A material id | Material id of shape. Use Structural 3D default materials as a guide. |
tip
To add multiple shapes or to use S3D.SB.runGSD
, set "run_solve": false
.
S3D.SB.buildCustomShape
function#
Sample input for the S3D.SB.buildCustomShape
S3D.SB.buildCustomShape
function#
Sample response for the S3D.SB.importDXF
#
Build a Section via DXF data. Simply pass in the DXF in text format to build the Section. We strongly recommend importing your DXF via the Section Builder UI to test your DXF files first. Use these inputs as the basis of your options.
Key | Type | Description |
---|---|---|
data | string | DXF data in string format |
opts | object | Options to import DXF • cutout_shapes - if any of the polygons generated are cutouts, they will need to be defined by adding them to this array;• merge_tol - Merge points within this distance.;• clear_previous - Clear previous shapes with this DXF section;• translate_to_origin - Translate to Origin;• plane - Plane that DXF importer will use as reference. |
S3D.SB.save
#
Save and load user-defined custom sections to cloud storage. After building your Section using one of the SB functions, you can save it to your My Sections storage for use in future projects.
Key | Type | Description |
---|---|---|
Name | string | User-defined section name |
S3D.SB.solve
#
Submit section and calculate section properties. This may already be included in some functions such as S3D.SB.buildCustomShape
where the run_solve
property has been set to true
.
Key | Type | Description |
---|---|---|
manual_J | string | (Optional) If J cannot be solved, user should provide a J value to use for analysis. |
info
Section should already be set via one of the earlier functions.
S3D.SB.submit
#
Submit section to your S3D structural model
Key | Type | Description |
---|---|---|
section_id | integer | Section ID for this section to be submitted to your structural model |
manual_J | string | (Optional) If J cannot be solved, user should provide a J value to use for analysis. |
info
Section should already be set via one of the earlier functions.
S3D.SB.runGSD
#
After a section (and units system too) is set via S3D.SB.buildCustomShape
, further analysis may be performed on the section using the General Section Designer (GSD). This uses FEA to calculate the capacity of reinforced concrete sections. Provide loads, reinforcement and select from material databases to run FEA analysis and receive basic pass/fail results for complex concrete sections.
Key | Type | Accepts | Description | Default |
---|---|---|---|---|
design_code | string | ACI 318 or ACI , AS 3600 , BS , CSA , EN , IS 456 , NSCP 2015 or PH | Design code used to select material properties. | |
concrete_class | string | • ACI 318: C2500 , C3000 , C3500 , C4000 , C4500 , C5000 , C6000 , C7000 , C8000 , C9000 , C10000 • AS: N20 , N25 , N32 , N40 , N50 , N65 , N80 , N100 , N120 • BS: C15 , C20 , C25 , C30 , C35 , C40 , C45 , C50 , C55 , C60 • CSA: C25 , C30 , C35 , C40 , C45 , C50 • EN: C20/25 , C25/30 , C30/37 , C35/45 , C40/50 , C45/55 , C50/60 , C20/25 , C60/75 , C70/85 , C80/95 , C90/105 • IS456: M15 , M20 , M25 , M30 , M35 , M40 , M45 , M50 , M55 , M60 • NSCP 2015: C3000/20.68 , C3500/24.13 , C4000/27.68 , C4500/31.03 , C5000/34.47 , C6000/41.36 | Concrete Class Code - this will determine the values used for Young's Modulus and Stress/Strain data of the concrete. | |
steel_grade | string | • ACI 318: Grade 40 , Grade 50 , Grade 60 , Grade 75 • AS: R250N , D500N , D500L • BS: Grade 250 , Grade 460 , Grade 500 • CSA: 300R , 400R/W , 500R/W • EN: Class A , Class B , Class C • IS456: Grade 250 , Grade 415 , Grade 500 • NSCP 2015: Grade 230 , Grade 275 , Grade 415 | Steel Grade - this will determine the values used for Young's Modulus and Stress/Strain data of the steel reinforcement. | |
base_point | [object] | An object | An object contains the properties z , y for the point where forces applyed to the section. If undefined - point is located at section centroid. | |
isNominal | boolean | true , false | Set this prop true if you need to calculate limit forces using only characteristic material strength and without strength reduction factor(phi). | false |
reinforcement | [object] | An array of objects | An array of objects where each object contains the properties z , y and diam for reinforcement position and diameters. | |
loads | [object] | An array of objects | An array of objects where each object contains design loads. | |
return_stress_results | boolean | true , false | Set this to true for detailed results of the mesh and material stresses/strains. | false |
S3D.SB.runGSD
function#
Sample input for the S3D.SB.runGSD
S3D.SB.runGSD
function#
Sample response for the The following image is an example of a complex section that may be analyzed using GSD.
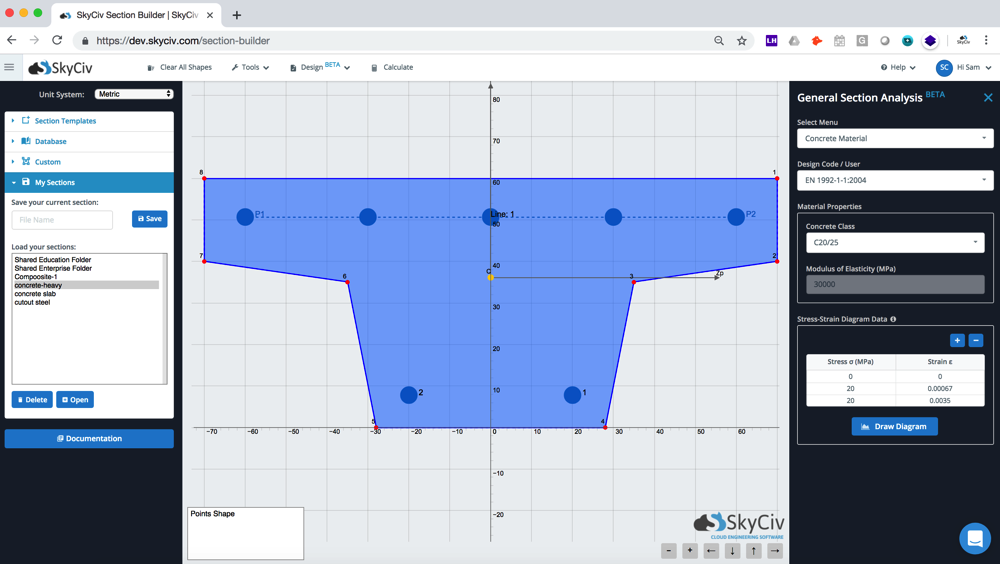