Creating the API Object
#
API ObjectIn this example we are creating the entire payload object by supplying the following components:
The
auth
objectThe
options
objectThe
functions
array - an array of objects defining which functions to run[ {...}, {...}, ... ]
Starting a session - using function
S3D.session.start
Setting the model - using function
S3D.model.set
Running an analysis - using function
S3D.model.solve
important
Our packages offer a simplified way to achieve the following code. View the various packages here.
View this Sample in Action
s3d_model
#
Sample The model data is the JSON formatted object you create that describes your structure. The following is an example of a simple beam.
If the S3D.file.save
function is used, this model will be available on SkyCiv's S3D platform for later viewing.
The following image is a visual representation of what the JSON model object above will create.
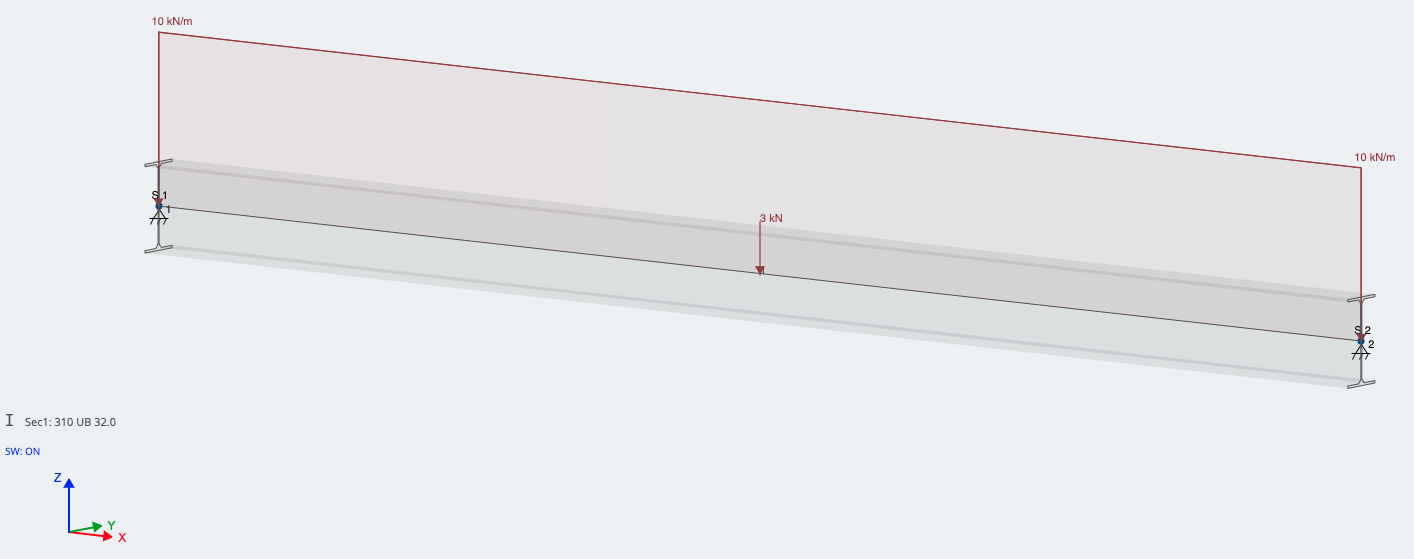