S3D Integration
You can automatically integrate your calculation pack with S3D, by writing a s3d_integration.js
file. The purpose of this file is to extract data out of your model and analysis results, to run your calculations off. If your calc pack has a s3d_integration.js
file and your calculate.js
follows the standardised format, your solution will appear in the list in S3D:
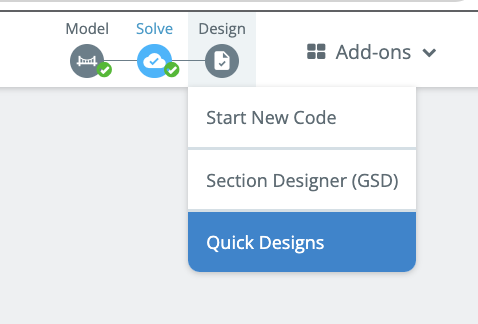
From here, your model will be auto-integrated with the following UI:
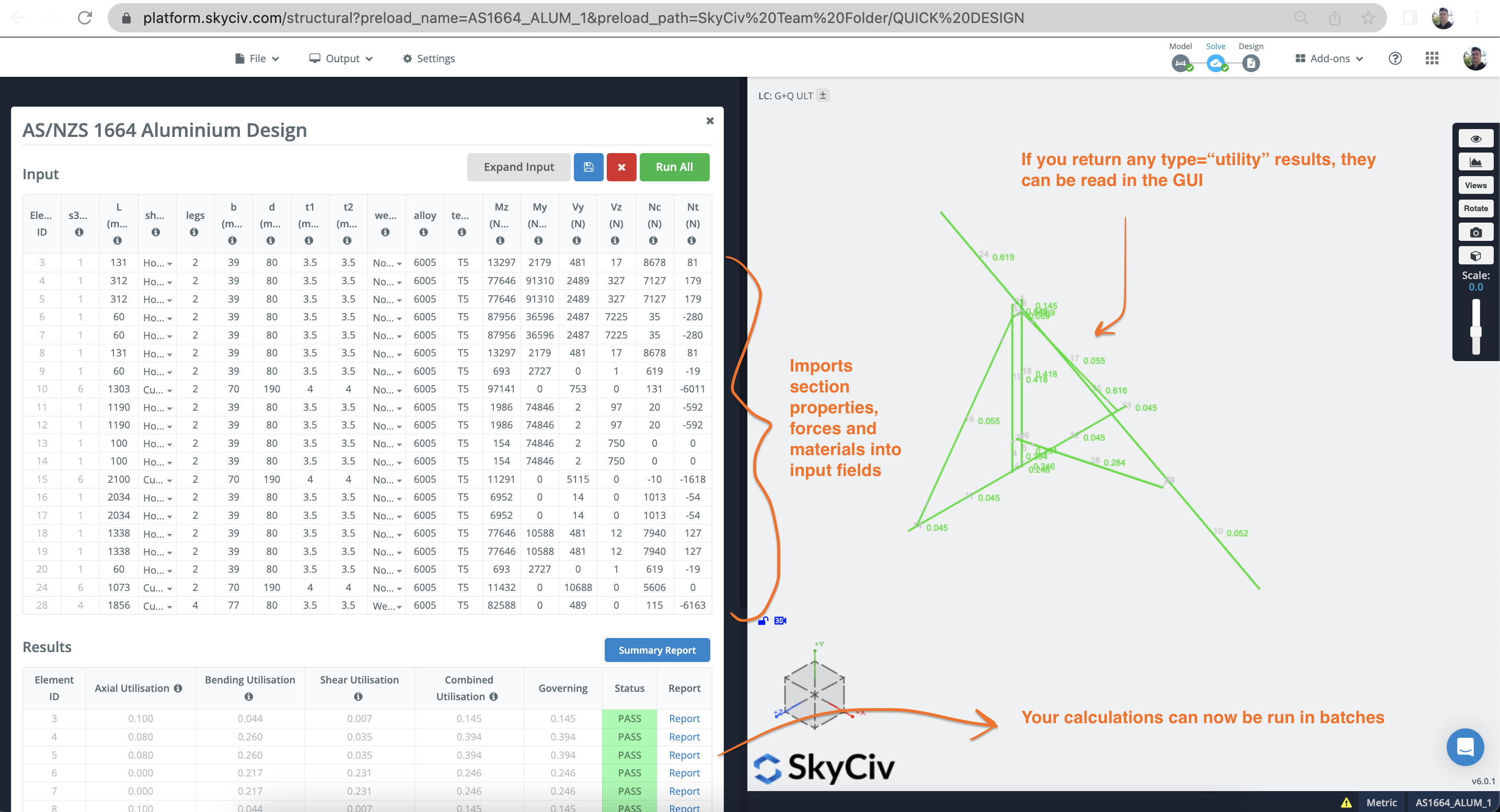
#
Layoutimportant
This file uses the UI format for S3D! So for instance, use s3d_model.elements
not s3d_model.members
!
Use this file to compile an array of inputs for your calculator, that can be then run in batches:
#
Helper FunctionsStructureHelpers.getDesignForces()
#
Returns an array of design forces for each member. It will scan all load combos and determine the worst result for each member in both + and - directions.
Output:
tip
For members that are rigid links, getDesignForces
will return no design forces.
StructureHelpers.getMemberLength()
#
Returns the member's length, including if offsets are used
StructureHelpers.ezDesignForces()
#
The ezDesignForces helper is an alternative to StructureHelpers.getDesignForces()
. This method transforms and returns a simplified analysis object that is simpler to use in quick design. Only actions in action_list are retrieved. For shorter run-time when sections are not transformed in section builder and when loading inside of the individual load combinations is not of interest, use the express method. For all other cases, use the detailed method.
Express method : Use include_lc = false
. Individual values at each element station are not retrieved and only maximum and minimum values are retrieved from each load combination. This only works when no transformations exist in sections, because transformations must be addressed at the individual load stations. An error will be thrown if one of the sections has a transformation applied (rotation
, mirror_y
or mirror_z
) in section builder.
Detailed method: Use include_lc = true
. Results at each station are retrieved for each member and for each load combination. Output loads are applied in line with geometric axis of members as they appear in section builder with no transformations (rotations or mirrors). Usually, this means the strong flexural axis is about the horizontal axis Z and the weak flexural axis is about the vertical axis Y.
#
ParametersKey | Type | Accepts | Description |
---|---|---|---|
s3d_analysis | object | S3D.results.getAll(true) | Global S3D analysis object |
s3d_model | object | S3D.model_data | Global S3D model object |
action_list | array | An array of actions to retrieve from the analysis_result object, includes: ["axial", "bmd_y", "bmd_z", "sfd_y", "sfd_z", "torsion"] | Multiple actions possible, see S3D.results.getAll(true)[0] for the full list. If no value is given, the list following list of internal forces will be used: action_list = ["axial", "bmd_y", "bmd_z", "sfd_y", "sfd_z", "torsion"] |
include_lc | boolean | true , false | If true , includes individual load combinations and section transformations. If false , includes envelope results neglecting section transformations: |
include_lc = false
)#
Example (Express Method include_lc = true
)#
Example (Detailed Method UnitHelpers.convert()
#
Convert units from one system to another. This is very useful when trying to control the inputs coming into your calculation.js file. For instance, say someone is using a model that is in ft, but your calculator only works for mm
, you can use UnitHelpers.convert( member_length , s3d_units.length, "mm");
to take it from whatever system the s3d_model is and translate that input to mm
#
LoggerThe global logger()
function is available to add to the quick design logs
#
WarningsThe global warn()
function is available to notify users of warning when running calculations
#
ExampleBelow is an example of a more advanced integration for aluminimum design. It takes in the s3d_model and results, in order to compile an array of inputs. It imports the member's length, section properties and design forces.
#
Formatting ResultsYou can control the S3D Results table with the following options
Key | Value |
---|---|
s3d_symbol | The heading to show in the S3D Results table. |
s3d_info | The info tip to show in the S3D Results table. |
#
How to Test CodeTo test your code, first start by creating a Draft. Then take the UID of that draft and run the following code in your S3D console:
This will open up the module on the left panel and run your latest s3d_integration.js
file on the server.