Overview
SkyCiv Apps allow you to build your own App directly on top of SkyCiv Structural 3D. We have provided access to an extensive list of S3D functions to help you create custom tools.
You can activate various built in apps in your account settings. Once they have been toggled on, you will find them available in the Apps dropdown menu inside of S3D.
Below is the Bill of Materials App - one of the built in apps. This App gathers and displaus useful insights about the current model such as materials, sections, cost and more!
caution
Apps should use functions only from the S3D Functions category.
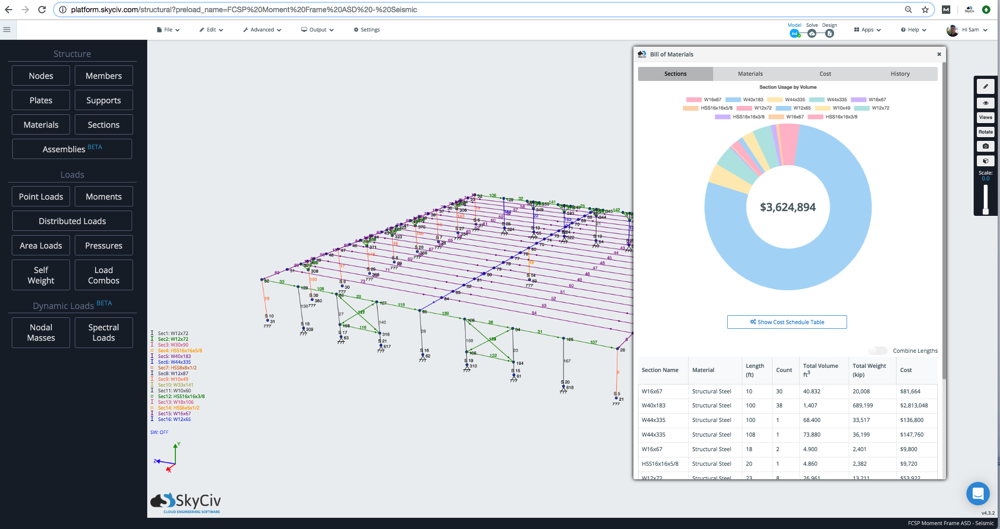
#
Getting StartedTo get started, simply open up SkyCiv Structural 3D. Then open your developer console by hitting CTRL+SHIFT+J
or F12
(this may depend on your browser) and pasting the below code. This will install a sample app, you can continually copy and paste code into the Console.
#
Initialising OptionsKey | Type | Description |
---|---|---|
id | string | File name of your model. |
name | string | Path in your cloud file storage. |
content | string | HTML string of what should be displayed in the app. |
width | string | Optional width setting for app window. |
height | string | Optional height setting for app window. |
icon_img | string | URL of image to be used. |
icon_img_square | string | URL of image to be used within the app header. |
draggable | bool | Is the app window draggable. True by default. |
resizable | bool | Can user resize window? Used alongside resizeableFunction to update. |
resizeableFunction | function | Function which runs when the app window is resized. |
help_url | string | URL of documentation or help page. |
onInit | function | Function which runs when the page loads the app. |
onFirstOpen | function | Runs when the app is open for the first time. |
onSoftwareUpdate | function | Function to run after changes are made to your model in S3D. |
beforeShow | function | Before the app is shown, run this function. |
aferShow | function | After the app is shown, run this function. |
beforeHide | function | Before the app is hidden, run this function. |
aferHide | function | After the app is hidden, run this function. |
#
Core FunctionsOnce your app is initalized, you can call the following functions to make the most of your app. SKYCIV_APPS.create()
will create the app and add it to SKYCIV_APPS
as an object. You can then access all of the default and user defined functions for this app.
In the above example, we created an app. We now have access to the following functions from the console:
#
What's next?Once you've built and tested your app, email us at [email protected] to learn more about getting this installed on our platform for internal or public use!